Here are five best practices for working with React:
1.Use functional components whenever possible: Functional components are simpler and easier to understand than class-based components, and they can often do everything that class-based components can do. For example:
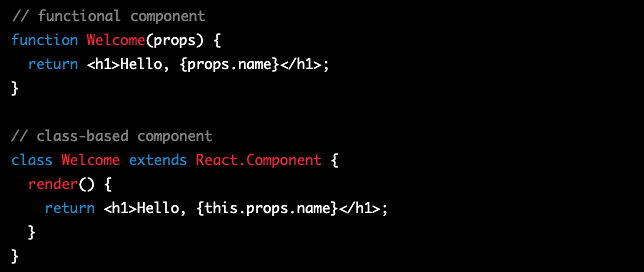
2. Use the useEffect
hook for side effects: The useEffect
hook allows you to perform side effects (such as API calls or subscribing to events) in functional components. It is a safer and more efficient alternative to the componentDidMount
, componentDidUpdate
, and componentWillUnmount
lifecycle methods. For example:
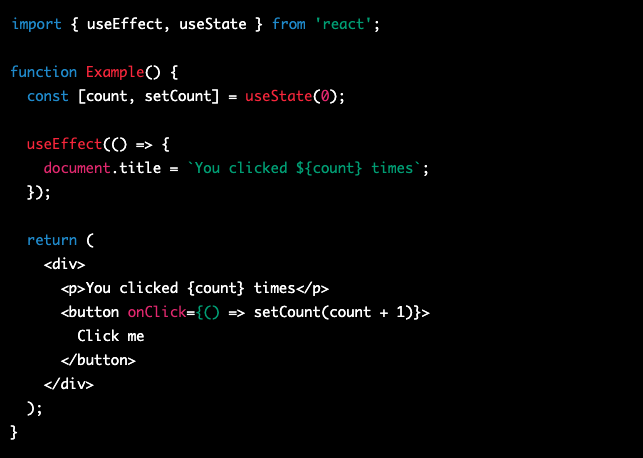
3. Use the useMemo
hook for performance optimization: The useMemo
hook allows you to memoize the result of expensive calculations so that they are only recalculated when the input dependencies change. This can improve the performance of your application by avoiding unnecessary re-renders. For example:
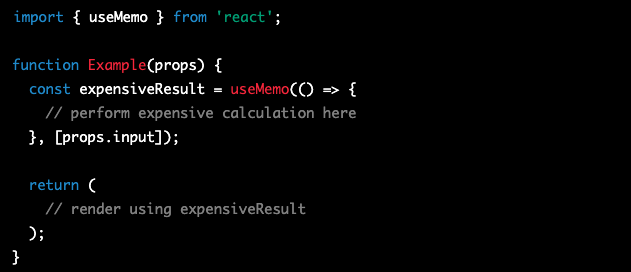
4. Use the useCallback
hook to avoid unnecessary re-renders: The useCallback
hook allows you to return a memoized callback function that will not change unless one of its dependencies changes. This can be useful for avoiding unnecessary re-renders when passing callback props to child components. For example:
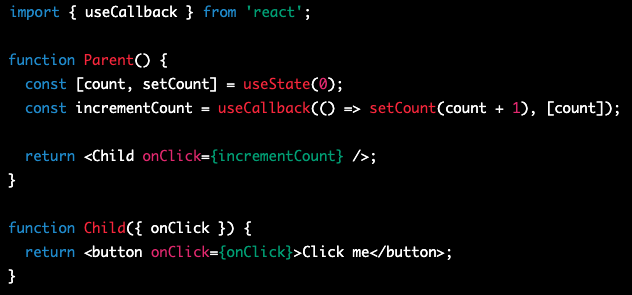
5. Use the React.Fragment
component to avoid unnecessary DOM nodes: The React.Fragment
component allows you to return multiple elements from a component without adding an extra DOM node. This can be useful for keeping your DOM structure clean and avoid issues with CSS or other DOM manipulation. For example:
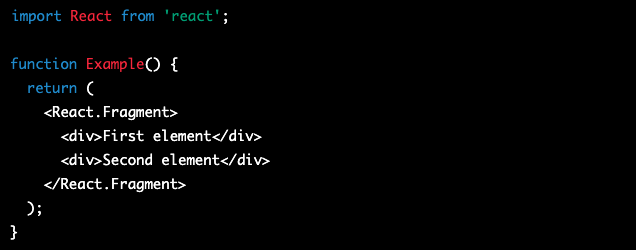